
Now we'ra going to set up a simple scene. We'll add two teapots to the scene, creating a hierarchy. Sounds difficult? Hell no!
First we have to add a camera, like this:
// We store a reference to the graphic manager
SDE.Graphic.Manager gMan = SDE.Engine.Instance.GraphicManager;
// We create a 3D camera
SDE.Graphic.Camera3 cam = gMan.CreateCamera3D();
Everything you can do with the engine can be accessed from SDE.Engine which is a singleton, so you can call SDE.Engine.Instance to use it. Remember, never create an engine's object explicitely with new operator. In this case, we want to use the Graphic Manager only. We create a Camera3 so that we can render 3D objects.
Now we create two objects, two teapots in this example:
SDE.Graphic.Node parentTeapot = gMan.CreateTeapot();
SDE.Graphic.Node childTeapot = gMan.CreateTeapot();
We're going to establish a hierarchy between them. The parent teapot will be positioned at (-1,0,0) and the child teapot will be positioned at (1,0,0) . The child teapot's center is it's parent position, so while the parent's position will be (-1,0,0) the child's position should be (2,0,0) so that the final position will be (-1,0,0) + (2,0,0) = (1,0,0).
// Object setup
parentTeapot.AddChild(childTeapot);
parentTeapot.Position = new Vector3(-1,0,0);
childTeapot.Position = new Vector3(2,0,0);
We need to create two materials. One material will be red and the other will be blue. Why we need materials? Without materials, the engine doesn't renders the object, because it doesn't know HOW to render the object: with a color, with a texture, whatever. So we create two materials and asign each to a teapot:
// Material creation
parentTeapot.Material = gMan.Core.CreateMaterial("red");
childTeapot.Material = gMan.Core.CreateMaterial("blue");
Finally we have to set up the camera:
// Camera setup
cam.Position = new Vector3(0,0,-3);
cam.Target = new Vector3(0,0,0);
cam.Background = System.Drawing.Color.DarkGreen;
cam.AddToScene(parentTeapot);
gMan.AddCamera(cam);
It'll be positioned at (0,0,-3) and will look at (0,0,0). We'll set the background color to DarkGreen. Now we have to add the objects to the camera's scene. If you don't add the objects to the camera's scene, they wont be rendered. If you remove the parent teapot, nothing will be rendered. If you remove the child teapot, only the parent will be rendered. So you can add and remove objects to the hierarchy to show or hide them.
We have to add the camera to the engine so that it would be rendered. In the next tutorials, we will use different cameras to achieve pretty nice techniques.
This tutorial was a little complicated, but don't worry, you can read the tutorial one more time just to be sure you understand all the concepts. Believe me, it will save you a lot of time later. Oh! you can now run the app and see... wow! two teapots!
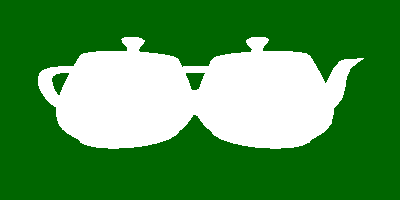
But, why are both white? That's got an easy answer. This objects are automatically generated by DirectX for testing purposes, so colors or textures won't be used. But you still have to asign them a material or they won't be rendered at all!